일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 자바
- 스프링
- 1과목
- 코딩봉사
- C++
- 정보처리산업기사
- BFS
- programmers
- 회고
- softeer
- 파이썬
- MYSQL
- 공부일지
- CJ UNIT
- 코딩교육봉사
- python
- 시나공
- 백준 알고리즘
- 알고리즘
- 프로그래머스
- SW봉사
- 데이터베이스
- SQL
- 문제풀이
- 소프티어
- 백준알고리즘
- kotlin
- java
- 백준
- 코틀린
Archives
- Today
- Total
JIE0025
[Testcode][슬라이스][Controller] CategoryControllerTest 본문
728x90
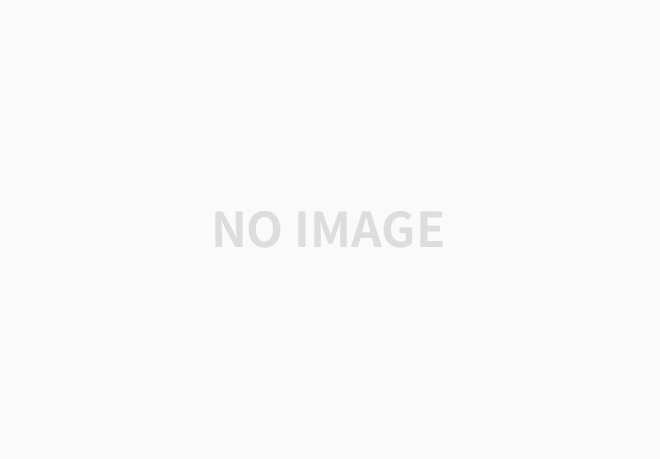
컨트롤러 단에서 서비스/레포지토리를 거치지 않는, 컨트롤러 자체를 위한 테스트 코드이다.
이 경우, 컨트롤러에 요청이 잘 들어오고 응답이 잘 거치면 되는지 테스트해주면 되기 때문에 CRUD 네가지를 체크해주었다.
package com.FlagHome.backend.domain.category.controller;
import com.FlagHome.backend.domain.category.dto.CategoryPatchDto;
import com.FlagHome.backend.domain.category.dto.CategoryPostDto;
import com.FlagHome.backend.domain.category.dto.CategoryResultDto;
import com.FlagHome.backend.domain.category.entity.Category;
import com.FlagHome.backend.domain.category.mapper.CategoryMapper;
import com.FlagHome.backend.domain.category.service.CategoryService;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.gson.Gson;
import org.hamcrest.Matchers;
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
import org.mockito.Mockito;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.boot.test.mock.mockito.MockBean;
import org.springframework.http.MediaType;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.ResultActions;
//import org.springframework.test.web.servlet.request.MockMvcRequestBuilders.;
import java.util.ArrayList;
import java.util.List;
import static org.hamcrest.Matchers.is;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.ArgumentMatchers.startsWith;
import static org.mockito.BDDMockito.given;
import static org.mockito.Mockito.when;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
@SpringBootTest
@AutoConfigureMockMvc
public class CategoryControllerTest {
private final String DEFAULT_URL = "/api/categories";
@Autowired
private MockMvc mockMvc;
@MockBean
private CategoryService categoryService;
@MockBean
private CategoryMapper mapper;
@Autowired
private Gson gson;
@Test
@DisplayName("[컨트롤러] 카테고리 추가 테스트")
public void createCategoryTest() throws Exception {
//given
CategoryPostDto categoryDto = CategoryPostDto.builder()
.koreanName("알고리즘").englishName("english").categoryDepth(0L).build();
String content = gson.toJson(categoryDto);
Category result = Category.builder().build();
result.setId(1L);
//mocking
given(mapper.CategoryPostDtoToCategory(any(CategoryPostDto.class), any(CategoryService.class))).willReturn(Category.builder().build());
given(categoryService.createCategory(any(Category.class))).willReturn(result);
//when //then
mockMvc.perform(
post(DEFAULT_URL)
.contentType(MediaType.APPLICATION_JSON)
.content(content)
.accept(MediaType.APPLICATION_JSON))
.andExpect(status().isCreated())
.andExpect(header().string("Location", is(Matchers.startsWith(DEFAULT_URL))));
}
@Test
@DisplayName("[컨트롤러] 카테고리 수정 테스트")
public void updateCategoryTest() throws Exception {
//given
CategoryPatchDto categoryPatchDto = CategoryPatchDto.builder()
.koreanName("알고리즘")
.englishName("algorithm")
.categoryDepth(0L).build();
Long categoryId = 1L;
String content = gson.toJson(categoryPatchDto);
Category result = Category.builder()
.koreanName("알고리즘")
.englishName("algorithm")
.categoryDepth(0L).build();
result.setId(categoryId);
//mocking
given(mapper.CategoryPatchDtoToCategory(any(CategoryPatchDto.class), any(CategoryService.class))).willReturn(Category.builder().build());
given(categoryService.updateCategory(any(Category.class))).willReturn(result);
//when//then
mockMvc.perform(
patch(DEFAULT_URL + "/" + categoryId)
.contentType(MediaType.APPLICATION_JSON)
.content(content)
).andExpect(status().isOk());
}
@Test
@DisplayName("[컨트롤러] 모든 카테고리 가져오기 테스트")
public void getCategoriesTest() throws Exception {
//given
List<CategoryResultDto> allList = List.of(
new CategoryResultDto("가가가","aaa",0L,null),
new CategoryResultDto("나나나","bbb",0L,
List.of(
new CategoryResultDto("다다다",
"ccc",1L,null)
)
)
);
//mocking
given(categoryService.getCategories()).willReturn(new ArrayList<>());
given(mapper.CategoryListToCategoryResultDtoList(any(List.class))).willReturn(allList);
//when //then
mockMvc.perform(
get(DEFAULT_URL)
.accept(MediaType.APPLICATION_JSON)
).andExpect(status().isOk())
.andExpect(jsonPath("$[0].koreanName").value(allList.get(0).getKoreanName()))
.andExpect(jsonPath("$[0].englishName").value(allList.get(0).getEnglishName()))
.andExpect(jsonPath("$[0].categoryDepth").value(allList.get(0).getCategoryDepth()))
.andExpect(jsonPath("$[1].koreanName").value(allList.get(1).getKoreanName()))
.andExpect(jsonPath("$[1].englishName").value(allList.get(1).getEnglishName()))
.andExpect(jsonPath("$[1].categoryDepth").value(allList.get(1).getCategoryDepth()));
}
@Test
@DisplayName("[컨트롤러] 카테고리 삭제 테스트")
public void deleteCategoryTest() throws Exception {
//given
Long categoryId = 1L;
//mocking
Mockito.doNothing().when(categoryService).deleteCategory(any(Long.class));
//when //then
mockMvc.perform(delete(DEFAULT_URL+"/"+categoryId))
.andExpect(status().isNoContent());
}
}
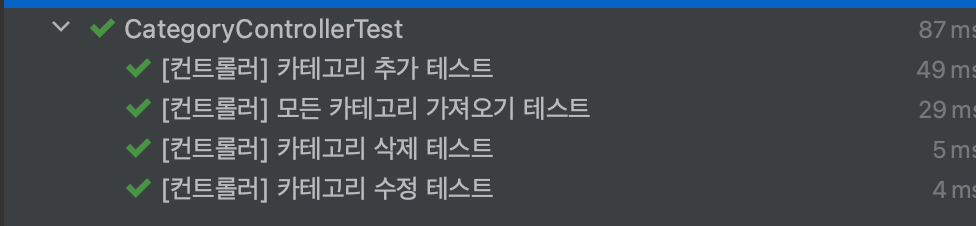
'Application > Test' 카테고리의 다른 글
[Testcode][통합] CategoryIntegrationTest (0) | 2023.01.09 |
---|---|
[Testcode][슬라이스][Service] CategoryServiceTest (0) | 2023.01.09 |
[Testcode][도메인][Entity] CategoryTest (0) | 2023.01.09 |
[Postman][5] PUT- 카테고리 수정(컨트롤러 요청메세지 테스트) (0) | 2022.12.20 |
[현재 상황 분석] 어디가 문제일지 & 앞으로 봐야할 부분들 찾기 (0) | 2022.12.19 |