일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 스프링
- 코틀린
- softeer
- 프로그래머스
- 자바
- programmers
- MYSQL
- CJ UNIT
- 백준 알고리즘
- 소프티어
- python
- SQL
- 정보처리산업기사
- 공부일지
- C++
- 시나공
- 파이썬
- java
- 백준
- SW봉사
- 코딩봉사
- 데이터베이스
- 백준알고리즘
- 코딩교육봉사
- 회고
- 문제풀이
- kotlin
- BFS
- 알고리즘
- 1과목
- Today
- Total
JIE0025
웹소켓 (WebSocket) 실시간 양방향 통신 (NodeJS 실습) 본문
✅ 웹소켓 프로토콜은 무엇이고 왜 사용할까?
개발을 할 때 HTTP 프로토콜을 이용하여 요청-응답 기반 단방향 통신을 했었다.
즉, 클라이언트에서 요청을 보내면, 서버는 응답을 반환 하고, 연결울 종료하는 방식이다.
이 경우 항상 클라이언트가 요청을 해야하며, 서버는 요청을 기다릴 수밖에 없다는 의미가 된다.
⚠️ HTTP 한계
- 만약 서버가 어떤 변경사항을 클라이언트에게 빠르게 알려줘야한다면 HTTP를 통해서 하기엔 어려워진다.
- HTTP 메시지에는 헤더가 차지하는 공간이 큰데, 채팅 앱 같이 단문의 메세지를 주고받는 경우 네트워크 낭비가 커질 수도 있다.
▶️ 웹소켓
이런 한계를 극복하기 위해 웹소켓 프로토콜이 나왔다.
클라이언트와 서버간 실시간 양방향 통신을 가능하게 한다.
양쪽에서 언제든지 메세지를 전달할 수 있다는 의미이다!
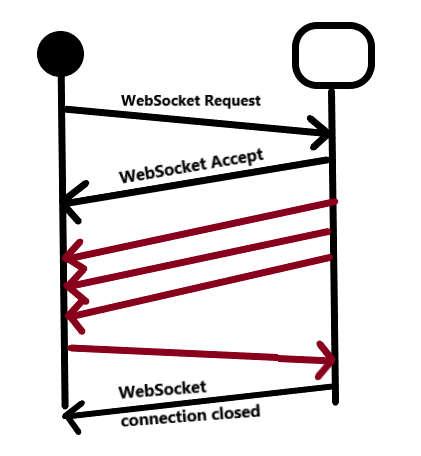
✅ 웹소켓 연결 과정 (Handshake)
1. 클라이언트는 서버로 GET 요청을 보낸다.
웹소켓에서만 사용되는 헤더 ( Connection, Upgrade )를 함께 보낸다
GET /chat HTTP/1.1
Host: www.test.com
Origin: www.test.com
Connection: Upgrade
Upgrade: websocket
Sec-WebSocket-Key: ...
Sec-WebSocket-Version: 13
2. 웹소켓 연결을 지원하는 서버일 때
101 Switching Protocols 상태코드를 응답한다.
HTTP/1.1 101 Switching Protocols
Upgrade: websocket
Connection: Upgrade
Sec-WebSocket-Accept: ...
3. 이제 웹소켓 클라이언트와 서버는 서로 양방향 통신할 수 있다.
✅ 서버
서버는 사용하는 언어/프레임워크에 따라 API가 다른데,
자바스크립트 NodeJS를 사용하여 서버를 구현할 땐 ws라는 npm패키지가 자주 사용된다.
ws, wss의 차이는 http, https와 같이 보안/신뢰성의 차이다.
server.js
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws) {
console.log('새로운 클라이언트 연결됨!');
ws.on('message', function incoming(message) {
console.log('받은 메시지:', message.toString());
// 모든 클라이언트에게 메시지 브로드캐스트
wss.clients.forEach(function each(client) {
if (client.readyState === WebSocket.OPEN) {
client.send(`서버에서 전달: ${message}`);
}
});
});
ws.send('서버에 연결되었습니다!');
});
✅ 클라이언트
가장 먼저 클라이언트는 WebSocket객체를 만들어야한다.
const socket = new WebSocket('ws://localhost:8080');
웹소켓 객체는 서버에서 발생하는 4종류의 이벤트가 있다.
- open : 서버와 연결을 맺음
- message : 서버에서 메세지를 받음
- error : 에러가 발생함
- close: 서버와 연결이 종료됨
socket.onopen = function () {
console.log('서버 연결 완료');
};
socket.onmessage = function (event) { //서버에서 메세지가 도착함
const li = document.createElement('li');
li.textContent = event.data;
document.getElementById('chat').appendChild(li);
};
클라이언트는 socket.send()를 이용하여 서버로 메세지를 보내줄 수 있따.
function sendMessage() {
const input = document.getElementById('message');
socket.send(input.value);
input.value = '';
}
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>WebSocket 채팅</title>
</head>
<body>
<h1>WebSocket 채팅</h1>
<input type="text" id="message" placeholder="메시지를 입력하세요">
<button onclick="sendMessage()">전송</button>
<ul id="chat"></ul>
<script>
const socket = new WebSocket('ws://localhost:8080');
socket.onopen = function () {
console.log('서버 연결 완료');
};
socket.onmessage = function (event) {
const li = document.createElement('li');
li.textContent = event.data;
document.getElementById('chat').appendChild(li);
};
function sendMessage() {
const input = document.getElementById('message');
socket.send(input.value);
input.value = '';
}
</script>
</body>
</html>
✅ 테스트

references
https://developer.mozilla.org/ko/docs/Web/API/WebSocket
WebSocket - Web API | MDN
WebSocket 객체는 WebSocket 서버 연결의 생성과 관리 및 연결을 통한 데이터 송수신 API를 제공합니다.
developer.mozilla.org
https://www.daleseo.com/websocket/
실시간 양방향 통신을 위한 웹소켓(WebSocket)
Engineering Blog by Dale Seo
www.daleseo.com
https://developer.mozilla.org/ko/docs/Web/API/WebSockets_API/Writing_WebSocket_client_applications
WebSocket을 이용하여 클라이언트 애플리케이션 작성하기 - Web API | MDN
WebSocket은 ws 프로토콜을 기반으로 클라이언트와 서버 사이에 지속적인 완전 양방향 연결 스트림을 만들어 주는 기술입니다. 일반적인 웹소켓 클라이언트는 사용자의 브라우저일 것이지만, 그렇
developer.mozilla.org
'Infra > Network' 카테고리의 다른 글
[FTP] 파일전송 프로토콜 (File Transfer Protocol) (0) | 2025.02.27 |
---|---|
포트(Port) 란 무엇이고 왜 있는걸까? (1) | 2024.12.06 |